Server routing is one of the most essential parts of web application development, especially scalability and performance. Node.js Server Routing Techniques matters because appropriate routing affects the performance and support of the application in the long term.
In this article we will show how to make routing optimal in Node.js not only for the performance’s sake but also for the support simplicity of the code. We will show what ways it can make your architecture more rational, structured and flexible.
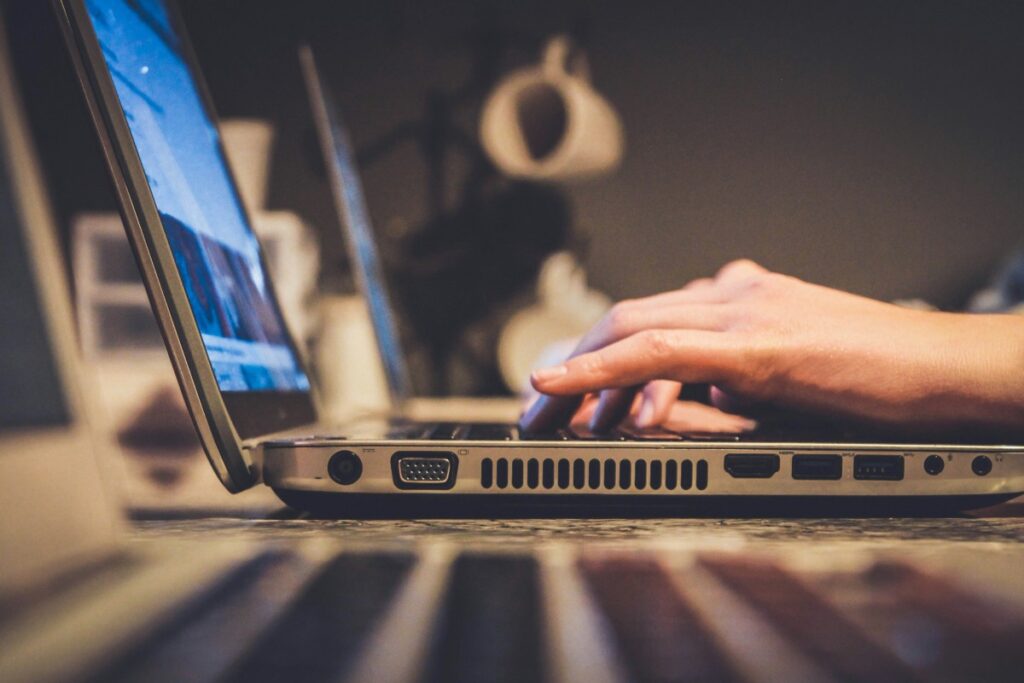
Proper routing facilitates better distribution of responsibilities among the system components, makes code easier to read and improves development speed, especially in collaborative projects. Efficient routing also has an impact on security, as it simplifies adding new operations and changing the application operation logic without compromising its integrity optimization.
Celadonsoft node development agency will show you the techniques that allow you to efficiently route and control data flows in an app and provide some code examples you can insert directly into your own projects.
Modular routing pattern with Express Router
When creating server applications in Node.js, not only is it required that the code be executing, but also that it be arranged in a manner where it will be readable and scalable. Of all the methods to improve the performance and structure of an application, one of the most significant is the use of a modular approach when organizing routes.
Why is this required?
Celadonsoft: “When the server app begins to expand, the disorderly definition of routes in a single file creates disorder and complication in tracking. Consider an API with hundreds of endpoints, all contained in server.js – the correct route or modifying it is a nightmare.”
express.Router() enables:
- Split the routes into functional modules (e.g., /users, /orders, /products).
- Facilitate code support and scaling.
- Enhance readability through structured logic.
How does it work?
For example, we have an API for users. Instead of writing all the routes in a single file, we’ll have a separate module for handling users:
Project structure:
//
/project-root
server.js
routes/
users.js
orders.js
controllers/
usersController.js
ordersController.js
//
routes/users.js:
//
const express = require('express');
const router = express.Router();
const usersController = require('./controllers/usersController');
router.get('/', usersController.getAllUsers);
router.post('/', usersController.createUser);
module.exports = router;
//
controllers/usersController.js:
//
exports.getAllUsers = (req, res) => {
res.json({ message: "User list" });
};
exports.createUser = (req, res) => {
res.json({ message: "User created" });
};
//
server.js (main application file):
//
const express = require('express');
const app = express();
const usersRoutes = require('./routes/users');
app.use('/users', usersRoutes);
app.listen(3000, () => {
console.log('Server running on port 3000');
});
//
Advantages of this approach
- Scalability: new routes are easy to add without congesting the main file.
- Clean code: one module manages its own part of the API.
- Ease of testing: routes and logic are separate, making tests simpler to write.
This approach makes the code more organized, reduces the technical overhead and makes developers’ lives easier. If you are building a scalable application, modular routing is something that needs to be done from the beginning.
Splitting logic: routes and controllers
In Node.js Server Routing Techniques, one of the concepts that allows you to keep your code clean and readable is the splitting of logic. Good application architecture assumes that routes are only responsible for managing HTTP requests, and all business logic is performed on separate controllers.
Why do we need it?
On small projects, you can see how all the query logic is dealt with right inside the routes.
//
app.get('/users', async (req, res) => {
const users = await db.getUsers();
res.json(users);
});
//
The code is working correctly, but if the application begins to increase and the routes amount to tens or hundreds, such an organization begins to wreak havoc. It is more difficult to introduce new functions, and re-use of the code is practically impossible.
How to organize the code correctly?
The best solution is to separate routes and controllers.
- Routes: they should only take the HTTP request and transfer control to the appropriate processor.
- Controllers: they deal with business logic, data processing and database interaction.
Example of segregation
Create a routes folder and file userRoutes.js:
//
const express = require('express');
const router = express.Router();
const userController = require('./controllers/userController');
router.get('/users', userController.getAllUsers);
module.exports = router;
//
Let’s create a controller in controllers/userController.js:
//
const db = require('./db');
exports.getAllUsers = async (req, res) => {
try {
const users = await db.getUsers();
res.json(users);
} catch (error) {
res.status(500). json({ message: 'server error' });
}
};
//
Advantages of this approach
- Cleanliness of the code: routes are not cluttered and easy to read.
- Testing convenience: controllers can be tested independently of routes.
- Reuse of logic: business logic can be easily reused in other parts of the application, for example, background processing tasks.
The separation of routes and controllers is not a recommendation, but standard practice for leading companies like Celadonsoft. This way, the code is easier to maintain, and the team will be able to complete their tasks more effectively.
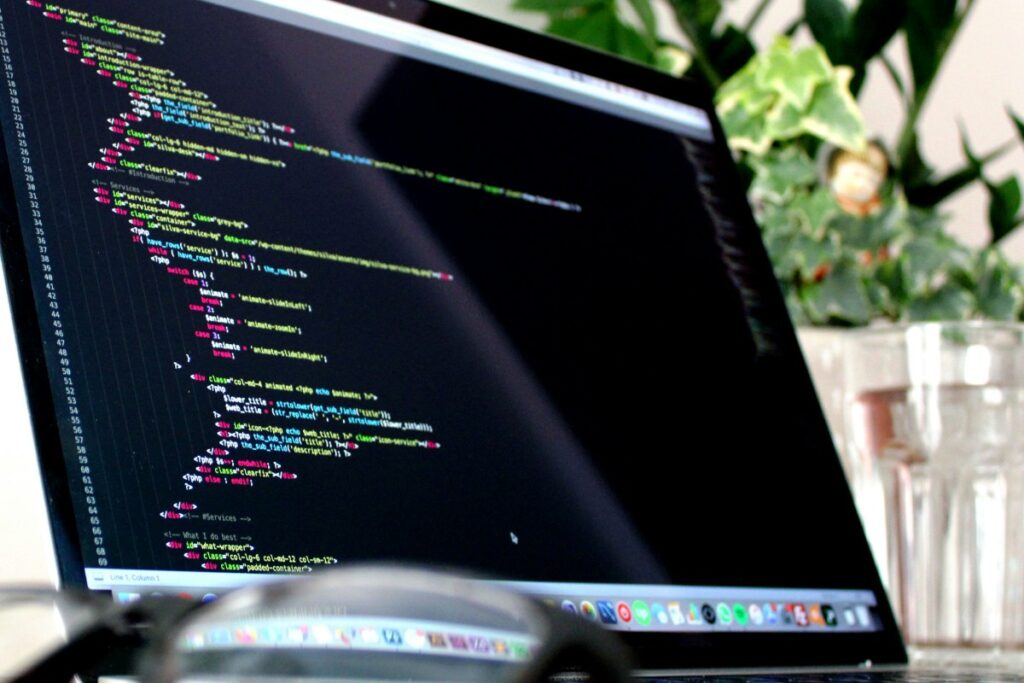
Using intermediate software (middleware) for reused logic
In today’s Node.js applications, the usability and scalability of the code is largely dependent on good use of middleware. This concept allows for the centralization of repetitive tasks such as authentication, logging, caching and error handling, eliminating redundant code routes and simplifying project support.
Why use middleware?
When developing a server application, it is inevitable that there are tasks to be performed for multiple routes. For example:
- Authentication – JWT token or API key verification before accessing protected routes.
- Request Logging – record information about incoming HTTP requests, which is useful for debugging and monitoring.
- Request frequency limitation (rate limiting) – prevents server overload or DDoS attacks.
- Data formatting and parsing – automatic processing of JSON-query numbers, compression of responses etc.
Middleware allows these processes to be carried out in separate functions and connected only where they are really needed.
How does it work with Express?
In Express middleware, these are the functions that receive the request object (req), the response object (res) and the next() function, allowing processing to be passed on further along the chain.
Example of basic middleware for query logic:
//
const loggerMiddleware = (req, res, next) => {
console.log(‘[${new Date().toISOString()}] ${req.method} ${req.url}’;
next(); // Give control to the following middleware or route handler
};
app.use(loggerMiddleware);
//
This middleware will run before each route handler and output information about the incoming request to the console.
Connecting middleware to specific routes
Not all middleware has to work globally. Sometimes it is necessary to limit their use only to certain routes.
Example middleware for authentication testing, used only on protected API endpoints:
//
const authMiddleware = (req, res, next) => {
const token = req.headers.authorization;
if (!token || token !== "secret token") {
return res.status(401). json({ message: "Unauthorized" });
}
next();
};
app.get("/private", authMiddleware, (req, res) => {
res.json({ message: "Welcome to the private route!" });
});
//
Middleware execution sequence
It is important to consider the order of the middleware connections, as Express performs them in the order they were announced. For example, if the middleware for error handling is declared to the primary route handler, it will not work correctly.
Correct order:
//
app.use(loggerMiddleware);
app.use(authMiddleware);
app.use(express.json());
app.get("/", (req, res) => res.send("Hello, world!"));
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500). json({ message: "Internal Server Error" });
});
//
Middleware is one of the most powerful tools in Express, allowing to separate logic, reduce code duplication and make server code more organized. With proper use of middleware, the readability, support, and scalability of the application can be greatly improved.
Rout group and prefixes to improve ordering
As your application grows, there will inevitably be more routes, and that will make for code anarchy. Useful route prefixes exist to introduce order into it, and the code will be improved in terms of readability and maintainability.
If you have an API for goods and users, it is a good idea to organize routes by functionality:
//
const express = require('express');
const app = express();
const userRoutes = require('./routes/users');
const productRoutes = require('./routes/products');
app.use('/api/users', userRoutes);
app.use('/api/products', productRoutes);
//
In this way, not only is request processing separated logically, but also API version handling is simple. For example, if you need to make changes, you can just create /api/v2/users without changing /api/v1/users.
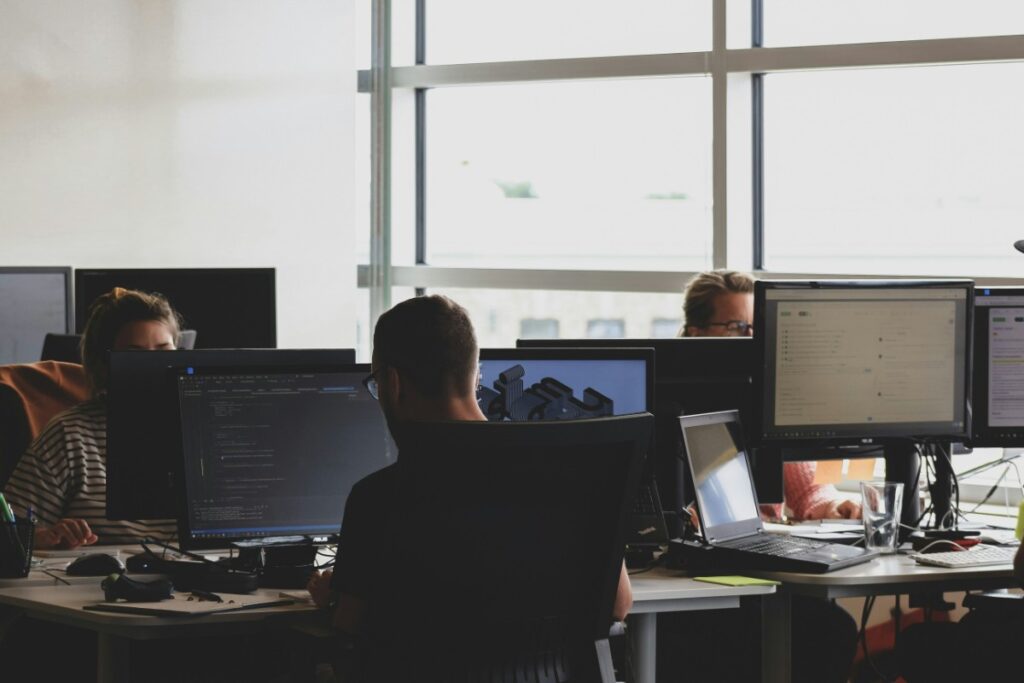
This greatly simplifies scaling and support for the application, especially in team environments where multiple developer teams are working on different aspects of the project.
Conclusion
Node.js Server Routing Techniques streamlining is not only a convenience issue, but among the most important scalability and code maintainability aspects. Proper route separation, middleware usage, error handling and API organization enable preventing mess in the code and making the application convenient for development and further maintenance. Furthermore, this makes the code easier to read, understand and debug for developers.
Implementing these best practices not only simplifies the day-to-day for the team, but also reduces the risks of scaling and introducing new functionality. By utilizing the best routing practices in Node.js, you lay the foundation for a strong, efficient and reliable server architecture.